LeetCode 104. Maximum Depth of Binary Tree - Python
Given a binary tree, find its maximum depth.
The maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node.
Note: A leaf is a node with no children.
Example:
Given binary tree [3,9,20,null,null,15,7],
3
/ \
9 20
/ \
15 7
return its depth = 3.
Solution 1 (Recursive) :
class Solution:
def maxDepth(self, root: TreeNode) -> int:
if not root:
return 0
return 1 + max(self.maxDepth(root.left), self.maxDepth(root.right))
Solution 2 (Recursive) :
class Solution:
def __init__(self):
self.max_depth = 0
def dfs(self, node: TreeNode, depth):
if not node:
return
if self.max_depth < depth:
self.max_depth = depth
if node.left:
self.dfs(node.left, depth + 1)
if node.right:
self.dfs(node.right, depth + 1)
def maxDepth(self, root: TreeNode) -> int:
if not root:
return 0
self.dfs(root, 1)
return self.max_depth
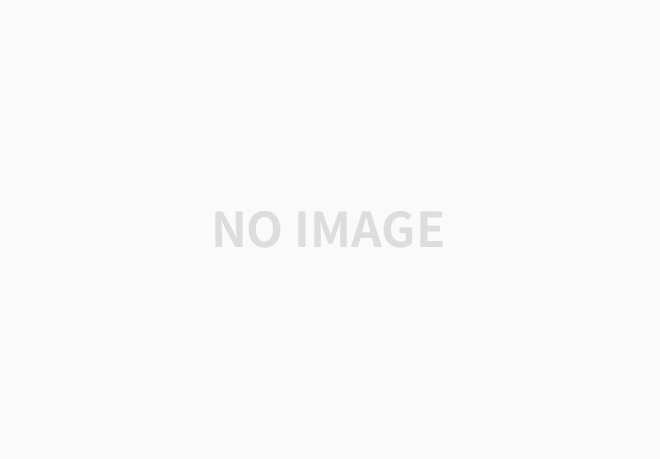
관련 개념 설명 :
'Python > PS in Python' 카테고리의 다른 글
LeetCode 226. Invert Binary Tree - Python (0) | 2020.08.09 |
---|---|
LeetCode 136. Single Number - Python (0) | 2020.08.06 |
LeetCode 617. Merge Two Binary Trees - Python (0) | 2020.08.03 |
[코드업 기초 100제] 1001~1099 (파이썬) - 문제 풀이용 (0) | 2020.07.28 |
[코드업 기초 100제] 1001~1099 (파이썬) (0) | 2020.07.28 |
댓글